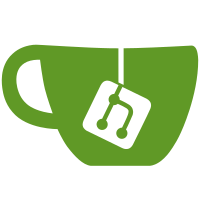
- Permit only decode token to get map details, - If user have token expired, set the token to null and reload the page. This feature will be updated when authentication stategy will be finished. Signed-off-by: Gregoire Parant <g.parant@thecodingmachine.com>
28 lines
1.0 KiB
TypeScript
28 lines
1.0 KiB
TypeScript
import { ADMIN_API_URL, ALLOW_ARTILLERY, SECRET_KEY } from "../Enum/EnvironmentVariable";
|
|
import { uuid } from "uuidv4";
|
|
import Jwt, { verify } from "jsonwebtoken";
|
|
import { TokenInterface } from "../Controller/AuthenticateController";
|
|
import { adminApi, AdminBannedData } from "../Services/AdminApi";
|
|
|
|
export interface AuthTokenData {
|
|
identifier: string; //will be a email if logged in or an uuid if anonymous
|
|
}
|
|
export const tokenInvalidException = "tokenInvalid";
|
|
|
|
class JWTTokenManager {
|
|
public createAuthToken(identifier: string) {
|
|
//TODO fix me 200d when ory authentication will be available
|
|
return Jwt.sign({ identifier }, SECRET_KEY, { expiresIn: "200d" });
|
|
}
|
|
|
|
public verifyJWTToken(token: string, ignoreExpiration: boolean = false): AuthTokenData {
|
|
try {
|
|
return Jwt.verify(token, SECRET_KEY, { ignoreExpiration }) as AuthTokenData;
|
|
} catch (e) {
|
|
throw { reason: tokenInvalidException, message: e.message };
|
|
}
|
|
}
|
|
}
|
|
|
|
export const jwtTokenManager = new JWTTokenManager();
|