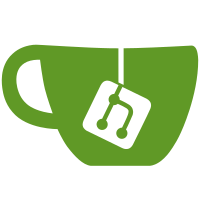
* Wrap websockets with HyperExpress * Add endpoints on pusher to resolve wokas * getting textures urls from pusher * Adding OpenAPI documentation for the pusher. The pusher now exposes a "/openapi" endpoint and a "/swagger-ui/" endpoint. * revert FRONT_URL * playerTextures metadata is being loaded via Phaser.Loader * fetch textures every time character or customize scene is open * Heavy changes: refactoring the pusher to always send the textures (and the front to accept them) * Sending character layer details to admin * Cleaning commented code * Fixing regex * Fix woka endpoints on pusher * Change error wording on pusher * Working on integration of the woka-list with the new admin endpoint. * Switching from "name" to "id" in texture object + using zod for woka/list validation * Add position on default woka data * Remove async on pusher option method * Fix woka list url * add options for /register * Fxiing loading the Woka list * Actually returning something in logout-callback * Copying messages to back too * remove customize button if no body parts are available (#1952) * remove customize button if no body parts are available * remove unused position field from PlayerTexturesCollection interface * removed unused label field * fix LocalUser test * little PlayerTextures class refactor * Fixing linting * Fixing missing Openapi packages in prod * Fixing back build Co-authored-by: Hanusiak Piotr <piotr@ltmp.co> Co-authored-by: David Négrier <d.negrier@thecodingmachine.com> * Add returns on pusher endpoints Co-authored-by: Alexis Faizeau <a.faizeau@workadventu.re> Co-authored-by: Hanusiak Piotr <piotr@ltmp.co> Co-authored-by: Piotr Hanusiak <wacneg@gmail.com>
43 lines
1.6 KiB
TypeScript
43 lines
1.6 KiB
TypeScript
import { ADMIN_API_TOKEN } from "../Enum/EnvironmentVariable";
|
|
import { stringify } from "circular-json";
|
|
import { parse } from "query-string";
|
|
import { socketManager } from "../Services/SocketManager";
|
|
import { BaseHttpController } from "./BaseHttpController";
|
|
|
|
export class DebugController extends BaseHttpController {
|
|
routes() {
|
|
this.app.get("/dump", (req, res) => {
|
|
const query = parse(req.path_query);
|
|
|
|
if (ADMIN_API_TOKEN === "") {
|
|
return res.status(401).send("No token configured!");
|
|
}
|
|
if (query.token !== ADMIN_API_TOKEN) {
|
|
return res.status(401).send("Invalid token sent!");
|
|
}
|
|
|
|
const worlds = Object.fromEntries(socketManager.getWorlds().entries());
|
|
|
|
return res.json(
|
|
stringify(worlds, (key: unknown, value: unknown) => {
|
|
if (value instanceof Map) {
|
|
const obj: any = {}; // eslint-disable-line @typescript-eslint/no-explicit-any
|
|
for (const [mapKey, mapValue] of value.entries()) {
|
|
obj[mapKey] = mapValue;
|
|
}
|
|
return obj;
|
|
} else if (value instanceof Set) {
|
|
const obj: Array<unknown> = [];
|
|
for (const [setKey, setValue] of value.entries()) {
|
|
obj.push(setValue);
|
|
}
|
|
return obj;
|
|
} else {
|
|
return value;
|
|
}
|
|
})
|
|
);
|
|
});
|
|
}
|
|
}
|